Part Four: Tracking Screens and Events in Adobe Experience Platform SDK using React Native
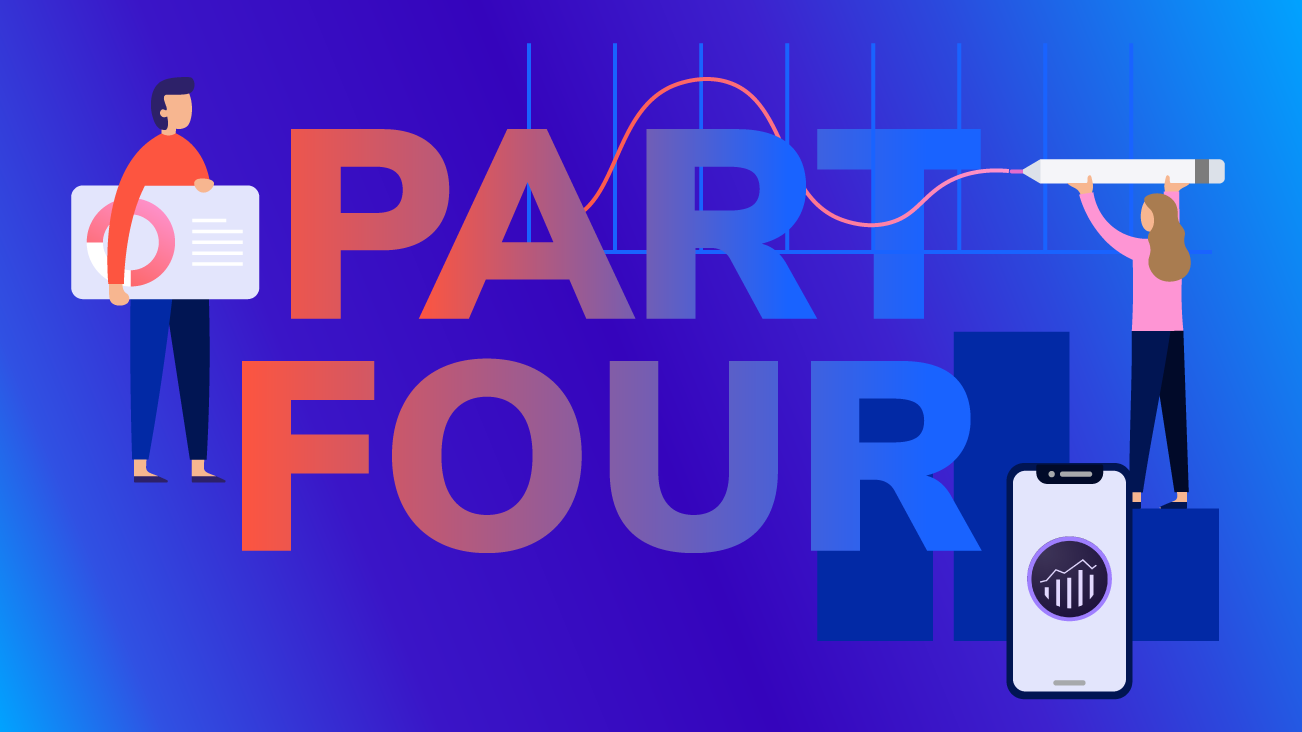
I have everything installed and working from parts one, two, and three, so I am ready to track user behavior. To do so I will need to track states (screens of the app, similar to pageviews on a website) and track actions (track specific events that take place inside the app). These functions come with the React Native ACPCore that I imported previously.
Track State
My app has two views. The main view that contains the items list and add button, and the modal that pops up when you are adding a new item. I am going to call track state in each of these components to register a screen view when each one is seen.
Notice there are two screens in my app:
In my main view component in App.js:
let contextData = {"key1": "some data", "key2": "another data point"}
ACPCore.trackState("Homepage", contextData);
In my modal component view:
let contextData = {"key1": "value1", "key2": "value2"}
ACPCore.trackState("AddGoalModal", contextData);
The trackState
function call on a screen view in an app is the equivalent of an s.t()
call on a page view of a website. If I look in the Charles Proxy network inspector, I can see these calls being sent from the simulator. Note that the state name (“AddGoalModal” for example) is populated as the page name (and will thus populate the pages/screens report) and any extra data I pass is sent as context data. This context data will need to be classified within Adobe Analytics.
Homepage screen view:
Modal screen view:
Track Action
There are three buttons in my app that I would like to track when they are pressed. I can do that with the trackAction
API. This is the equivalent of using s.tl()
calls on a website. My action name here is the custom link hit name.
In my AddNewGoal button click handler:
let buttonData = {"buttonKey": "buttonValue"}
ACPCore.trackAction("buttonPress", buttonData)
The Add New Goal button press beacon. Note the page event (pe) parameter is “lnk_o”:
Note that I am just sending extra data to illustrate that it is possible to do so. If you don’t need to send context data, you can omit it entirely from the API call. For example in my cancel handler:
ACPCore.trackAction("cancelAddGoal", {})
The Cancel button press hit:
Using Charles Proxy Tangent
Charles Proxy allows you to view all the HTTP traffic going on between the Internet and your computer. It is the best way to see the network requests that the phone simulator is sending to Adobe Analytics. Before diving into your requests, there’s some set up required in Charles.
In Charles, under the Proxy menu, select "SSL Proxy Settings." Add your tracking server and Launch domain here to ensure the hits are recorded correctly. If you do not set these settings, your hits may be blocked or time-out.
Charles and iOS Simulator
When using the iOS simulator, you will need to install the Charles SSL Root Certificate on your iOS simulator to avoid SSL errors. You can do that under Help > SSL Proxying > Install Charles Root Certificate in iOS Simulators.
Upon opening, Charles you should start to see a lot of network traffic. You can filter this traffic to only your tracking server using the filter at the bottom. That will make finding your analytics hits a lot easier.
Charles and Android Emulator
The process of setting up Charles to view your Android emulator traffic is a little more complicated and too long to cover here. It may require some trial and error also. If you get stuck, remember that that is always an option to start over with a fresh emulation device.
Also, it seems to be that when proxying Android emulator traffic through Charles, Charles needs to be open and running prior to running the React metro server. Otherwise, your app will not be able to communicate with the metro server and thus will not launch. I did not see this mentioned in any of the documentation, but it seemed to be the case for my development setup.
Here are some resources for setting up Charles and Android to view network requests made by your emulator:
- The Android Emulator and Charles Proxy: A Love Story
- Google Ads SDK Documentation for Charles Proxy
- Tealium Charles Proxy Android Documentation
Filtering traffic to just my tracking server in Charles (“omtr” in the filter):
LifeCycle Metrics
When viewing your hits in Charles, you’ll probably notice there is a lot more data being sent than just states and actions. Those are lifecycle metrics being sent to Adobe Analytics due to the ACPLifeCycle extension being registered. These hits are how you track things like app launches, app upgrades, and crashes amongst other events and dimensions. Read more on lifecycle metrics.
Lifecycle beacon:
Adobe Launch Rules
In my tracking calls above, I hardcoded some context data to be sent in with the beacon. I can accomplish the same thing without having to hardcode by using Launch. For example, in my Javascript cancel button handler I have:
ACPCore.trackAction("cancelAddGoal", {})
I can trigger a rule in Launch from this action with the following setting. I can also specify context data conditions for the action to get even more specific.
I add context data to be sent to Adobe Analytics:
After rebuilding the app, I can see the context data from Launch being sent in the beacon:
Conclusions
Each topic discussed in this series goes much deeper than I can cover in a few blog posts. I encourage you to peruse the official documentation of the tools I used here.